Testing Chat GPT 1o: the Strawberry Model
Credits: Galpy Creators, Open AI, Numpy, MatPlotLib, Gaia DR2, Pandas, Astropy, Matplotlib, Plotly, Pandas, Pytorch, Python

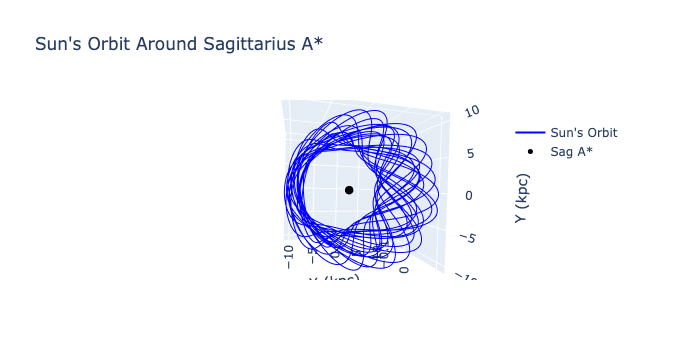
This Friday I spent some time experimenting with and testing GPT 1o, the new model from Open AI called Strawberry. I’ve been developing and analyzing large datasets using AI applications for the last year, instead of using google search for research for the blog. I’ve also been going to quite a bit of online school. Wow, there is much amazing information available now. So naturally I’ve gotten quite into physics. Specifically Astrophysics and the study of black holes, stars, and gravity. Recently I’ve been delving heavily into computational fluid dynamics. So Friday I decided to switch gears and learn about the orbital trajectories of celestial objets.
Here are the libraries that I imported. This will obviously grow:
import numpy as np
from galpy.orbit import Orbit
from galpy.potential import (PowerSphericalPotentialwCutoff, MiyamotoNagaiPotential,
NFWPotential, KeplerPotential)
from galpy.util import conversion
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D # For 3D plotting
from time import time
import plotly.graph_objects as go
import plotly.express as px
import pandas as pd
from tqdm import tqdm
from astropy import units
import torch
import torch.nn as nn
from torch.utils.data import TensorDataset, DataLoader
Here are the constants that I used. I focused GPT on specific angular momentum calculcations and used as much publicly available NASA data as possible. I computed Sagitarius A as a tensor for the sun’s orbital parameters and used machine learning on known data to forecast future positioning of the sun considering the gravitational potential of SAG. A. (the black hole at the center of the Milky Way) I have been working a lot with GPT to access public NASA API at pace to get neural networks running with data from the classic missions of the Voyagers, Juno, and the wonderful SDO and JWST. There are several well known galactic constants and constants specific to our sun contained here:
# Define Constants and Potentials
# ==========================
# Updated Galactic constants
ro = 8.178 # kpc, updated Sun-Galactic center distance
vo = 236.0 # km/s, updated circular velocity at solar radius
# Solar peculiar motion relative to LSR (Reid et al., 2020)
U_sun = 10.6 # km/s (towards Galactic Center)
V_sun = 10.7 # km/s (in direction of Galactic rotation)
W_sun = 7.6 # km/s (towards North Galactic Pole)
# Mass unit in galpy
mass_unit_msun = conversion.mass_in_msol(ro=ro, vo=vo)
# Masses in solar masses
mass_sag_a_star = 4.1e6 # Sag A* mass
disk_mass = 5e10 # Disk mass
bulge_mass = 1e10 # Bulge mass
# Convert masses to dimensionless amplitudes
amp_sag_a_star = -mass_sag_a_star / mass_unit_msun # Negative for attractive potential
amp_disk = disk_mass / mass_unit_msun
amp_bulge = bulge_mass / mass_unit_msun
# Create potentials for each component
# Bulge Potential
bulge = PowerSphericalPotentialwCutoff(
amp=amp_bulge,
alpha=1.8,
rc=1.9 / ro,
ro=ro,
vo=vo
)
# Disk Potential
disk = MiyamotoNagaiPotential(
amp=amp_disk,
a=3.0 / ro,
b=0.28 / ro,
ro=ro,
vo=vo
)
# Halo Potential using mvir and conc
halo = NFWPotential(
conc=15,
mvir=1.5, # In units of 1e12 solar masses
ro=ro,
vo=vo
)
# Sag A* Potential
sag_a_star_potential = KeplerPotential(
amp=amp_sag_a_star,
ro=ro,
vo=vo
)
# Combine potentials to create a custom Milky Way potential
custom_potential = [bulge, disk, halo, sag_a_star_potential]
# ==========================
# Define the Sun's Initial Conditions
# ==========================
# Normalize initial conditions
R_sun = 1.0 # Normalized units (ro)
vR_sun = 0.0 # Radial velocity in normalized units
vT_sun = 1.0 # Tangential velocity in normalized units
z_sun = 0.025 / ro # Normalize z_sun
vz_sun = 0.0 # Vertical velocity in normalized units
phi_sun = 0.0 # Galactic azimuthal angle
# Create the Orbit object for the Sun using normalized units
sun_orbit = Orbit(
vxvv=[R_sun, vR_sun, vT_sun, z_sun, vz_sun, phi_sun],
ro=ro,
vo=vo,
solarmotion=[U_sun, V_sun, W_sun]
)
# ==========================
# Define the Time Array and Convert Time Units
# ==========================
# Desired physical time span: 5 Galactic Years (each Galactic Year ≈ 230 million years)
orbital_period_Myr = 230 # Adjusted orbital period in million years
total_galactic_years = 1
years_per_galactic_year = orbital_period_Myr * 1e6 # Convert to years
total_physical_years = total_galactic_years * years_per_galactic_year # Total in years
# Number of time steps
num_steps = 10000
# Create a physical time array from 0 to total_physical_years
t_physical_years = np.linspace(0, total_physical_years, num_steps) # in years
# Calculate galpy's time unit in years
t_unit_Gyr = conversion.time_in_Gyr(ro=ro, vo=vo)
t_unit_years = t_unit_Gyr * 1e9 # Convert Gyr to years
# Convert physical time to galpy's internal time units
t_galpy = t_physical_years / t_unit_years # Dimensionless galpy time units
Matrix calculations become extremely important here, so NumPy’s utility really can’t be over-rated. The library is fast as a field mouse. The fact that this can all be put into one python script with the added PyTorch library to create. neural network… again in the same script… is somewhat mind boggling. Also I have to give a huge shout out to GalPy, whose library I explored extensively for this project. Excellent work on your website as well GALPy team!!
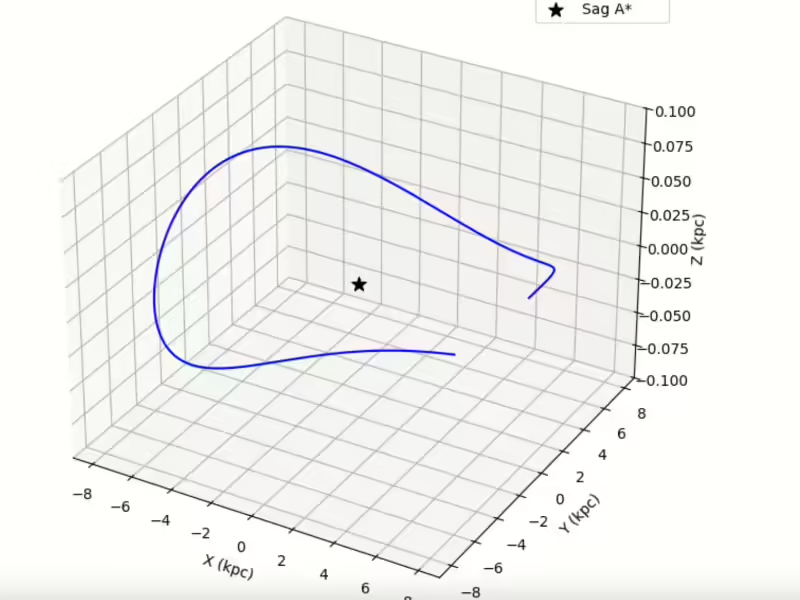
The Sun’s Orbital Path
So obviously this is rough, but I did use some extremely high quality NASA data to calculate this. Were talking voyager data from beyond. If anyone wants to peer review the code, I will happily send it to you in private, but I don’t want to share the entire machine learning code just yet. It definitely needs work.
This was about one orbital pass, and it would predict, ideally, the next orbit of the sun. But what about over time? Will the orbit shift significantly? Keep in mind that this is rough and that Sagittarius A will technically orbit the galactic center as well, because it is a relatively calm black hole.

Here is what a longer timescale would look like in the same model

20 Galactic Orbits of Sagittarius A forming an eye Torus.
Normalizations
Obviously this is a very rough calculcation, but if you take the average the 20 orbits computed here, you would at least have an orbital range of potential for the sun.
What’s missing?
The other large objects in the galaxy need to be accounted for to properly understand how these masses interact, because of the size of Sagittarius A. Although we do orbit it, our sun’s orbit is more irregular and honestly strange.
Top 10 Largest Objects in the Milky Way
Some of the largest objects in the Milky Way include (by mass):
1. Sagittarius A* (included in the simulation as a spiral bar black hole centrally located)
• Type: Supermassive Black Hole
• Mass: ~4,000,000 × Solar Masses (4 × 10⁶ M☉)
• Notes: As the supermassive black hole at the center of the Milky Way, Sagittarius A* has an immense gravitational pull, especially in its immediate vicinity.
2. Omega Centauri
• Type: Globular Star Cluster
• Mass: ~4,000,000 × Solar Masses (4 × 10⁶ M☉)
• Notes: This is the largest globular cluster associated with the Milky Way. Its combined mass of approximately 4 million solar masses results in a significant gravitational potential.
3. KY Cygni
• Type: Red Hypergiant Star
• Mass: ~30 × Solar Masses (30 M☉)
• Notes: One of the most massive known stars, KY Cygni’s substantial mass contributes to a strong gravitational field.
4. VY Canis Majoris
• Type: Red Hypergiant Star
• Mass: ~30 × Solar Masses (30 M☉)
• Notes: Another massive hypergiant, VY Canis Majoris has a gravitational potential comparable to KY Cygni.
5. Mu Cephei
• Type: Red Supergiant Star
• Mass: ~25 × Solar Masses (25 M☉)
• Notes: With a considerable mass, Mu Cephei exerts a strong gravitational influence.
6. VV Cephei A
• Type: Binary Star System (Supergiant Star)
• Mass: ~23 × Solar Masses (23 M☉)
• Notes: As part of a binary system, the combined mass enhances its gravitational potential.
7. V354 Cephei
• Type: Red Supergiant Star
• Mass: ~20 × Solar Masses (20 M☉)
• Notes: A massive supergiant contributing significantly to its local gravitational field.
8. Betelgeuse
• Type: Red Supergiant Star
• Mass: ~10–20 × Solar Masses (10–20 M☉)
• Notes: One of the most well-known stars, its mass varies within the given range, affecting its gravitational potential accordingly.
9. KW Sagittarii
• Type: Red Supergiant Star
• Mass: ~15 × Solar Masses (15 M☉)
• Notes: A massive star with a notable gravitational influence.
10. Antares
• Type: Red Supergiant Star
• Mass: ~12–15 × Solar Masses (12–15 M☉)
• Notes: While slightly less massive than some others on this list, Antares still has a significant gravitational potential.
So the next questions becomes, what can we make of the Omega Centauri Globular Star Cluster?
Hubble’s Zoom into Omega Centauri
The results are incredible! Hubble has some great footage of the stellar nursery:
Check out the video link to Hubble zooming in on the Omega Centuri area here:
A Great Example of RedShift and BlueShift
You can see all of the red stars and blue stars; the red stars are moving away and the blue stars are moving towards us. These are the actually distortions of light that Einstein predicted due to the maximum speed of light creating delays in the wavelengths that reach us.
Omega Centauri might also contain a black hole. James Webb should be looking at this area of the sky to try to figure out what is happening in the denser regions of this potential gravity well and black hole.
The motion of the stars in Omega Centauri
There is another most excellent video on the movement of the stars in this nebula; at a certain point of zooming in, there is an obvious average distance that becomes apparent and you can see how the stars orbit over time; the next steps in an analysis like this would be to find relationships between their movements.
Motion of stars in Omega Centauri
Dialing in the math and Calculus
This is the part of the script that I am going to work on the most for the next round. There are a few constants that I would like to full compute. They are listed in the graphs below:

Why is the James Webb Telescope so exciting?
The James Webb offers a completely new spectrum (heh) of technology to explore the inner galaxy’s dynamics. Here’s what perplexity had to say about it:
The James Webb Space Telescope (JWST) could be used to study Sagittarius A* in several important ways:
- 1. Infrared observations: JWST’s powerful infrared capabilities allow it to peer through the dust and gas obscuring the galactic center, providing clearer views of Sagittarius A* and its immediate surroundings.
- 2. Simultaneous multi-wavelength observations: JWST can observe in both near-infrared and mid-infrared wavelengths simultaneously, which has never been done before for Sagittarius A*. This allows scientists to better distinguish between the black hole and its flares.
- 3. Continuous monitoring: Unlike Hubble, which can only observe in 45-minute chunks as it orbits Earth, JWST can provide continuous observations of Sagittarius A* for extended periods.
- 4. Flare detection: JWST will spend about 25 hours observing Sagittarius A*, increasing the chances of capturing flares or other variable activity from the black hole.
- 5. Complementing Event Horizon Telescope (EHT) observations: JWST observations will be coordinated with the EHT campaign, providing valuable additional data to enhance our understanding of Sagittarius A*.
- 6. Studying the environment: JWST can observe the gas and stars in the vicinity of Sagittarius A*, helping scientists understand the black hole’s interactions with its surroundings.
- 7. High-resolution imaging in infrared: JWST’s advanced optics and infrared sensitivity could potentially provide more detailed images of the region around Sagittarius A*.
Here are more ways that the JWST will add to our knowledge of celestial objects in the Milky Way:
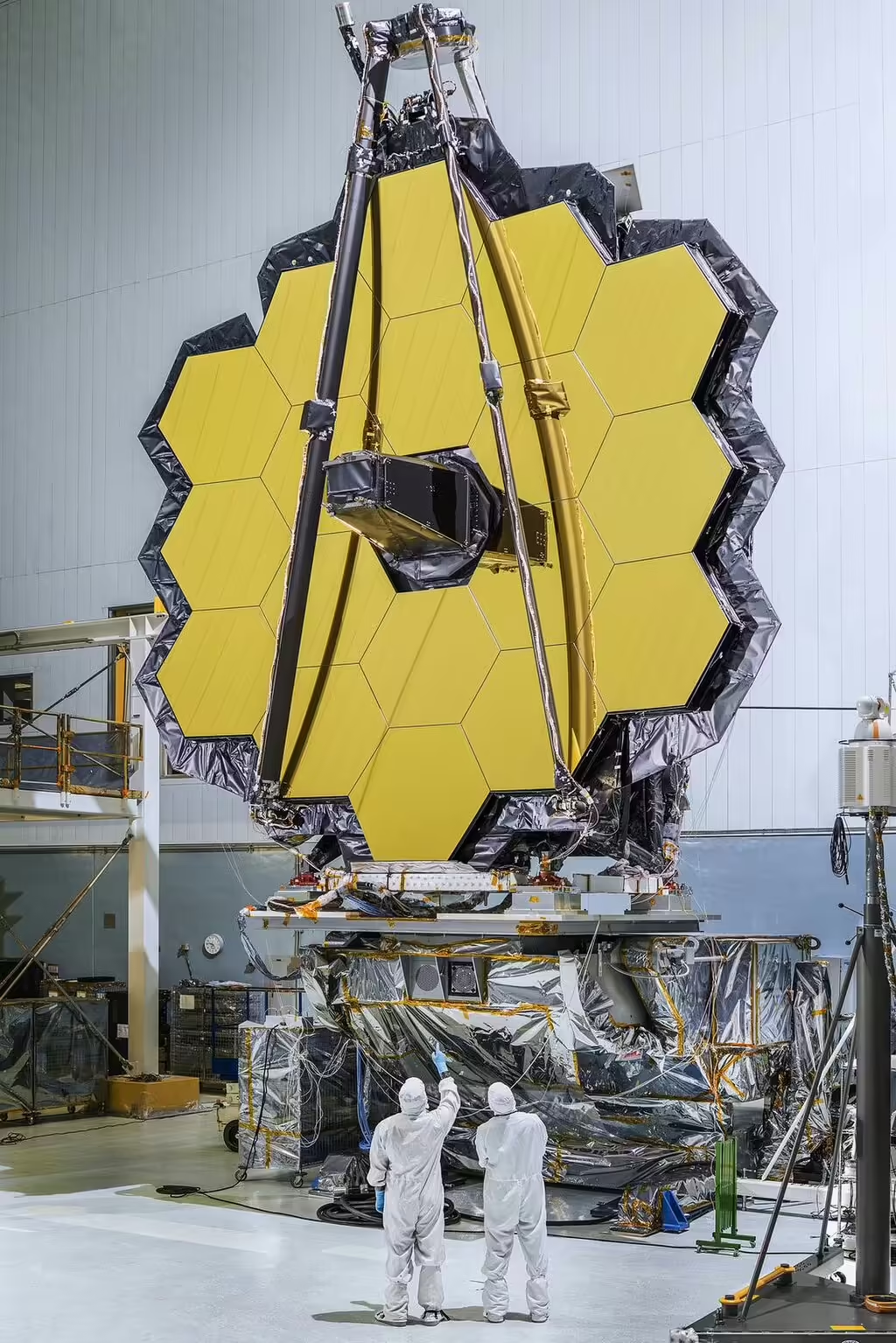
1. VY Canis Majoris
Type: Red Hypergiant Star
JWST Contributions:
• Infrared Imaging and Spectroscopy: VY Canis Majoris is surrounded by extensive dust and gas due to its significant mass loss. JWST’s Near-Infrared Camera (NIRCam) and Mid-Infrared Instrument (MIRI) can peer through this dust, allowing astronomers to study the star’s structure, mass-loss processes, and circumstellar environment in unprecedented detail.
• Temporal Monitoring: JWST can monitor changes in the star’s brightness and spectral features over time, providing insights into pulsations, eruptions, and variability associated with hypergiant stars.
2. Omega Centauri
Type: Globular Star Cluster
JWST Contributions:
• Resolved Stellar Populations: Omega Centauri contains millions of stars. JWST’s high-resolution imaging can resolve individual stars, especially those in crowded regions, enabling detailed studies of stellar evolution, age distribution, and metallicity variations within the cluster.
• Infrared Observations: Many stars in Omega Centauri may be obscured by interstellar dust. JWST’s infrared capabilities allow for the detection and analysis of cooler stars, such as red giants and white dwarfs, enhancing our understanding of the cluster’s diverse stellar populations.
• Exoplanet Studies: By observing transits and other exoplanetary phenomena within the cluster, JWST can contribute to the search for exoplanets in dense stellar environments.
3. Sagittarius A*
Type: Supermassive Black Hole at the Galactic Center
JWST Contributions:
• Infrared Monitoring of Surrounding Stars: Sagittarius A* is enshrouded in dust, making infrared observations essential. JWST can track the orbits of stars near the black hole with high precision, refining measurements of its mass and the dynamics of the surrounding stellar environment.
• Accretion Disk Studies: JWST can observe the accretion processes and any surrounding material interacting with Sagittarius A*, providing insights into black hole growth and behavior.
• Transient Phenomena: JWST can detect and study flares or other transient events caused by interactions near the event horizon, shedding light on high-energy processes in the vicinity of the black hole.
4. Betelgeuse
Type: Red Supergiant Star
JWST Contributions:
• Surface and Atmosphere Mapping: JWST’s high-resolution imaging can map Betelgeuse’s surface features, such as large convective cells, and study its extended atmosphere to understand mass loss mechanisms.
• Dust Production: Betelgeuse produces significant amounts of dust. JWST can analyze the composition and distribution of this dust, informing models of dust production in evolved stars.
• Future Supernova Insights: Understanding Betelgeuse’s current state helps predict its eventual supernova explosion. JWST’s observations can provide critical data on the pre-supernova conditions of red supergiants.
5. Antares
Type: Red Supergiant Star
JWST Contributions:
• Stellar Winds and Mass Loss: Similar to Betelgeuse, Antares experiences substantial mass loss. JWST can characterize the properties of its stellar wind and the surrounding circumstellar medium.
• Binary Interactions: If Antares has a binary companion, JWST can study the interactions between the stars, such as mass transfer or wind collision zones, which are essential for understanding the evolution of massive binaries.
• Chemical Composition: Infrared spectroscopy can reveal the chemical elements present in Antares’ atmosphere and ejected material, providing insights into nucleosynthesis in massive stars.
6. KW Sagittarii
Type: Red Supergiant Star
JWST Contributions:
• Detailed Spectroscopy: JWST’s NIRSpec (Near-Infrared Spectrograph) can perform high-resolution spectroscopy to determine the chemical abundances, temperature structures, and velocity fields within KW Sagittarii.
• Circumstellar Environment: Observing the dust and gas surrounding KW Sagittarii helps in understanding the processes driving mass loss and shaping the star’s immediate environment.
• Stellar Evolution Models: Data from JWST can refine models of red supergiant evolution, particularly for stars at the upper mass range.
7. VV Cephei A
Type: Binary Star System (Supergiant Star)
JWST Contributions:
• Binary Interaction Studies: JWST can observe the interactions between the two stars in the system, such as mass transfer, wind collisions, and orbital dynamics, which are critical for understanding the evolution of massive binary systems.
• Spectral Analysis: Using JWST’s spectroscopic instruments, astronomers can dissect the individual contributions of each star, revealing their temperatures, compositions, and wind properties.
• Eclipsing Events: If the binary system experiences eclipses, JWST can monitor these events to gain insights into the sizes, shapes, and atmospheres of the component stars.
8. Mu Cephei
Type: Red Supergiant Star
JWST Contributions:
• Mass Loss Mechanisms: JWST can study the processes driving Mu Cephei’s mass loss by analyzing its stellar wind and circumstellar dust through infrared observations.
• Surface Dynamics: High-resolution imaging can reveal surface features and convection patterns, enhancing our understanding of the dynamics in red supergiant atmospheres.
• Chemical Enrichment: By examining the ejected material, JWST can assess how Mu Cephei contributes to the chemical enrichment of the interstellar medium.
9. KY Cygni
Type: Red Hypergiant Star**
JWST Contributions:
• Extended Atmosphere Studies: JWST can probe the extended atmospheres of KY Cygni, revealing temperature gradients, density structures, and shock waves that influence mass loss.
• Dust Formation: Understanding where and how dust forms around KY Cygni helps in piecing together the life cycles of massive stars and their role in seeding the galaxy with heavy elements.
• Variability Monitoring: JWST can track changes in brightness and spectral features, providing data on pulsations and episodic mass-loss events.
10. V354 Cephei
Type: Red Supergiant Star**
JWST Contributions:
• Infrared Observations of Dust: JWST can characterize the dust composition and distribution around V354 Cephei, offering clues about the star’s mass-loss history and future evolution.
• Stellar Wind Analysis: By studying the infrared emission from stellar winds, JWST can determine the velocity, density, and temperature of the outflowing material.
• Temporal Studies: Monitoring V354 Cephei over time with JWST can reveal changes in its atmosphere and mass-loss rate, informing models of red supergiant behavior.
General Advantages of JWST for Studying Massive Stars and Related Objects
1. Infrared Sensitivity:
• Many of the objects listed are enshrouded in dust, which absorbs visible light but is transparent to infrared. JWST’s ability to observe in the infrared allows astronomers to penetrate these dusty regions and obtain clearer images and spectra.
2. High Spatial Resolution:
• JWST’s large aperture and advanced optics provide high-resolution images, enabling the detailed study of stellar surfaces, circumstellar environments, and crowded star clusters like Omega Centauri.
3. Spectroscopic Capabilities:
• Instruments like NIRSpec and MIRI allow for detailed spectroscopic analysis, which is essential for determining chemical compositions, temperature structures, and kinematics of celestial objects.
4. Temporal Monitoring:
• JWST can conduct repeated observations over time, facilitating the study of variability, transient events, and dynamic processes in massive stars and their environments.
5. Synergy with Other Observatories:
• JWST’s observations can complement data from other telescopes (e.g., ground-based observatories, the Hubble Space Telescope) to provide a more comprehensive understanding of these objects.
Specific Use Cases and Research Opportunities
• Stellar Evolution: By studying massive stars at different life stages with JWST, we can test and refine our models of galactic evolution and growth, particularly for red supergiants and hypergiants that end their lives as supernovae. There is so much to learn!
• Mass Loss and Feedback: Understanding how massive stars lose mass and interact with their surroundings is crucial for comprehending their role in galactic ecology. JWST’s observations can quantify mass-loss rates and the impact on the interstellar medium.
• Black Hole Environments: For Sagittarius A*, JWST can provide insights into the population of stars and gas near the black hole, influencing theories about galactic center dynamics and black hole feeding mechanisms.
• Star Cluster Dynamics: In Omega Centauri, JWST can explore the distribution and properties of its stars, shedding light on its origin—whether it’s a genuine globular cluster or the core of a merged dwarf galaxy.
These specific reasons make the James Webb telescope extraordinarily exciting. It’s important that we turn to the major tensors of our region of the galaxy to better understand our situation, so that perhaps, we can find more situations like ours out there. More life.
Some more epic pictures for reference to just how weird space is.

The Sagittarius A Galaxy amongst the Nebula, using simulations.
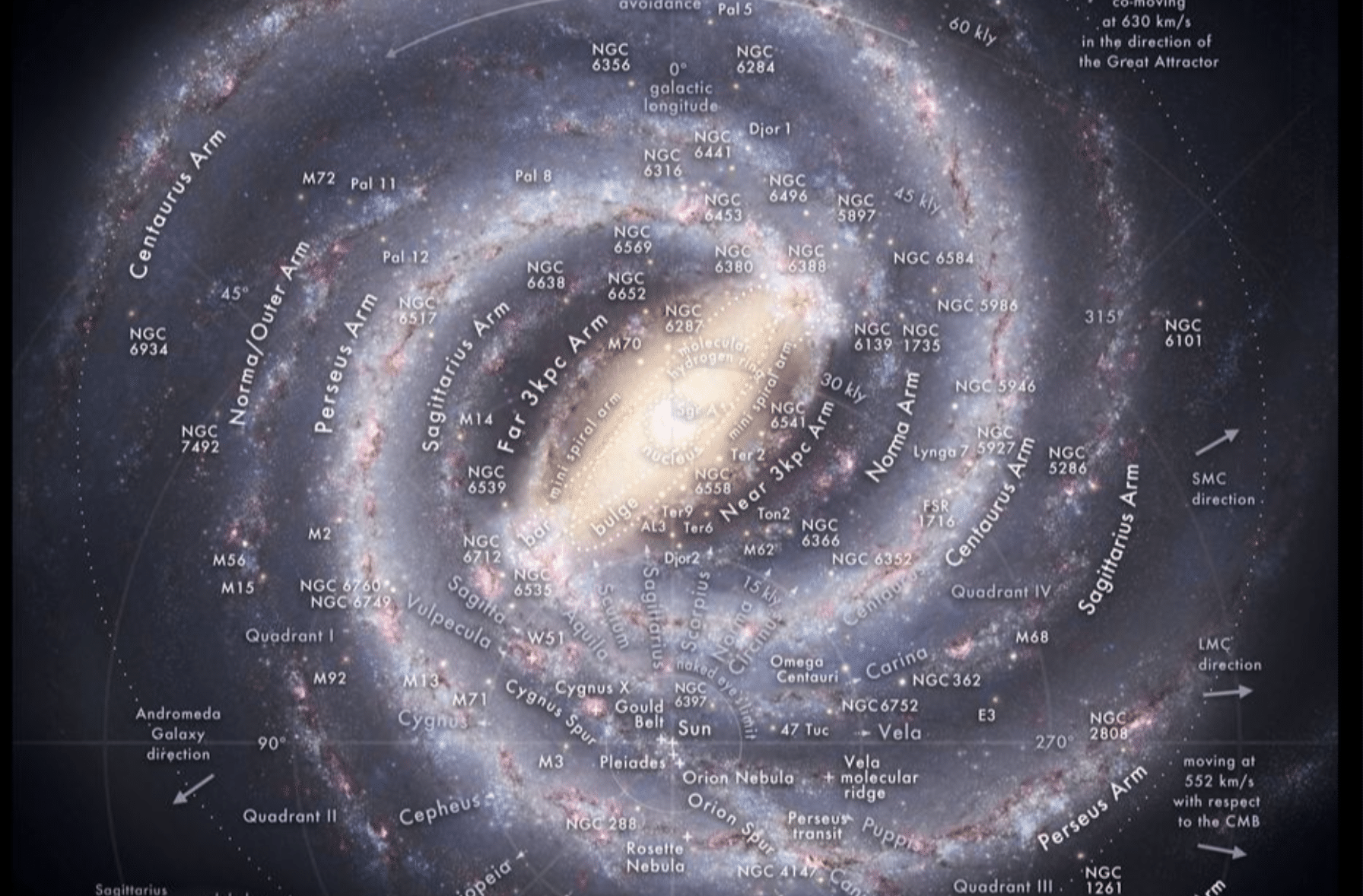
This map is 2D; in reality there would be a fairly significant amount of movement on the z axis for these objects, perhaps more than is currently accounted for or understood. I have more research to do on this, but my modeling seemed to suggest this (maybe its not?) it would appear that gravity works as a bubble would as we move through space; in other worlds gravity wells seem to have a tendency to be spherical! or at least circular. This might be a property of dark energy, as it appears to hold things together with a kind of negative pressure that exists beyond certain maxima of distance in gravity. Maybe dark energy particles are just extraordinarily small! We need more space stations, observatories and science facilities like LHC at CERN and LIGO to be able to really figure this stuff out. More AI. I’ll be doing another article on gravity waves here shortly. And hopefully soon here I can simulate a quasar! (I just need my 1o limit to reset cause I burned through it doing this analysis!)